- Published on
javadocの書き方
- Authors
- Name
- Kikusan
java.net.httpをサンプルとして見ていきます。
実際の中身はjava.internal.net.http
の実装クラスにありますが、公開されるインタフェースのjavadocとして見ます。
実装クラスについてはjavadocはほぼ書かれておらず、むしろ実装に関するコメントが多くありました。
また、基本的なjavadocの話はこちらの記事がよかったです。
アノテーション
タグ | 書き方 | 出力 |
---|---|---|
@author | @author Kikusan | 作成者: Kikusan |
@version | @version 1.0 | バージョン: 1.0 |
@see | @see <a href="*****">*****</a> @see class#method() | 関連項目: *****, class.method() |
@since | @since 11 | 導入されたバージョン: 11 |
@param | @param paramName 引数説明文 | パラメータ: paramName - 引数説明文 |
@return | @return 戻り値説明文 | 戻り値: 戻り値説明文 |
@throws | @throws MyException 例外説明文 | 例外: MyException - 例外説明文 |
@link | 内容は{@link #method()} を参照 | 内容はmethod()を参照 |
@code | {@code null} は例外を発生させます | null は例外を発生させます |
どのようになるかは下にある画像を参照
package-info
パッケージの責任を記載する。
https://docs.oracle.com/javase/jp/11/docs/api/java.net.http/java/net/http/package-summary.html
/**
* <h2>HTTP Client and WebSocket APIs</h2>
*
* <p> Provides high-level client interfaces to HTTP (versions 1.1 and 2) and
* low-level client interfaces to WebSocket. The main types defined are:
*
* <ul>
* <li>{@link java.net.http.HttpClient}</li>
* <li>{@link java.net.http.HttpRequest}</li>
* <li>{@link java.net.http.HttpResponse}</li>
* <li>{@link java.net.http.WebSocket}</li>
* </ul>
*
* <p> The protocol-specific requirements are defined in the
* <a href="https://tools.ietf.org/html/rfc7540">Hypertext Transfer Protocol
* Version 2 (HTTP/2)</a>, the <a href="https://tools.ietf.org/html/rfc2616">
* Hypertext Transfer Protocol (HTTP/1.1)</a>, and
* <a href="https://tools.ietf.org/html/rfc6455">The WebSocket Protocol</a>.
*
* <p> In general, asynchronous tasks execute in either the thread invoking
* the operation, e.g. {@linkplain HttpClient#send(HttpRequest, BodyHandler)
* sending} an HTTP request, or by the threads supplied by the client's {@link
* HttpClient#executor() executor}. Dependent tasks, those that are triggered by
* returned CompletionStages or CompletableFutures, that do not explicitly
* specify an executor, execute in the same {@link
* CompletableFuture#defaultExecutor() default executor} as that of {@code
* CompletableFuture}, or the invoking thread if the operation completes before
* the dependent task is registered.
*
* <p> {@code CompletableFuture}s returned by this API will throw {@link
* UnsupportedOperationException} for their {@link
* CompletableFuture#obtrudeValue(Object) obtrudeValue}
* and {@link CompletableFuture#obtrudeException(Throwable)
* obtrudeException} methods. Invoking the {@link CompletableFuture#cancel
* cancel} method on a {@code CompletableFuture} returned by this API may not
* interrupt the underlying operation, but may be useful to complete,
* exceptionally, dependent stages that have not already completed.
*
* <p> Unless otherwise stated, {@code null} parameter values will cause methods
* of all classes in this package to throw {@code NullPointerException}.
*
* @since 11
*/
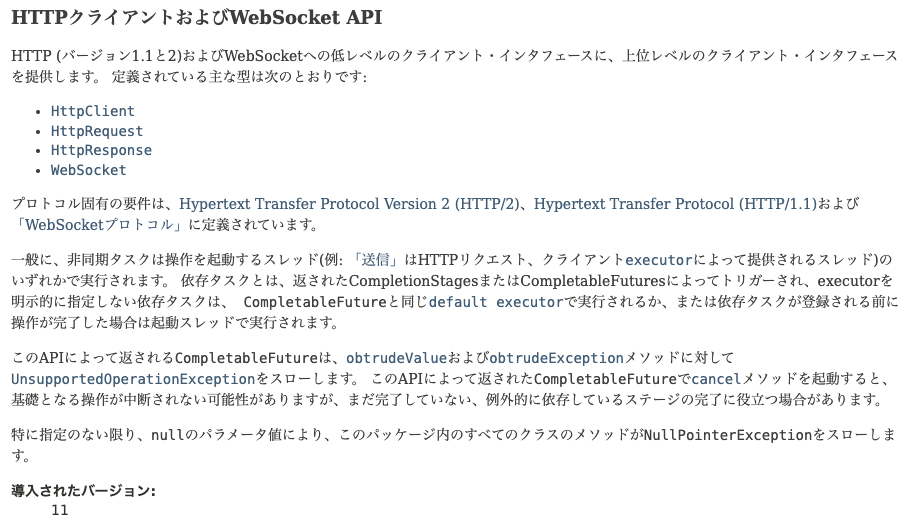
Class
クラスの責任を記載する。
基本的なクラスの使用方法と、注意点も記載している。
https://docs.oracle.com/javase/jp/11/docs/api/java.net.http/java/net/http/HttpClient.html
/**
* An HTTP Client.
*
* <p> An {@code HttpClient} can be used to send {@linkplain HttpRequest
* requests} and retrieve their {@linkplain HttpResponse responses}. An {@code
* HttpClient} is created through a {@link HttpClient#newBuilder() builder}. The
* builder can be used to configure per-client state, like: the preferred
* protocol version ( HTTP/1.1 or HTTP/2 ), whether to follow redirects, a
* proxy, an authenticator, etc. Once built, an {@code HttpClient} is immutable,
* and can be used to send multiple requests.
*
* <p> An {@code HttpClient} provides configuration information, and resource
* sharing, for all requests sent through it.
*
* <p> A {@link BodyHandler BodyHandler} must be supplied for each {@link
* HttpRequest} sent. The {@code BodyHandler} determines how to handle the
* response body, if any. Once an {@link HttpResponse} is received, the
* headers, response code, and body (typically) are available. Whether the
* response body bytes have been read or not depends on the type, {@code T}, of
* the response body.
*
* <p> Requests can be sent either synchronously or asynchronously:
* <ul>
* <li>{@link HttpClient#send(HttpRequest, BodyHandler)} blocks
* until the request has been sent and the response has been received.</li>
*
* <li>{@link HttpClient#sendAsync(HttpRequest, BodyHandler)} sends the
* request and receives the response asynchronously. The {@code sendAsync}
* method returns immediately with a {@link CompletableFuture
* CompletableFuture}<{@link HttpResponse}>. The {@code
* CompletableFuture} completes when the response becomes available. The
* returned {@code CompletableFuture} can be combined in different ways to
* declare dependencies among several asynchronous tasks.</li>
* </ul>
*
* <p><b>Synchronous Example</b>
* <pre>{@code HttpClient client = HttpClient.newBuilder()
* .version(Version.HTTP_1_1)
* .followRedirects(Redirect.NORMAL)
* .connectTimeout(Duration.ofSeconds(20))
* .proxy(ProxySelector.of(new InetSocketAddress("proxy.example.com", 80)))
* .authenticator(Authenticator.getDefault())
* .build();
* HttpResponse<String> response = client.send(request, BodyHandlers.ofString());
* System.out.println(response.statusCode());
* System.out.println(response.body()); }</pre>
*
* <p><b>Asynchronous Example</b>
* <pre>{@code HttpRequest request = HttpRequest.newBuilder()
* .uri(URI.create("https://foo.com/"))
* .timeout(Duration.ofMinutes(2))
* .header("Content-Type", "application/json")
* .POST(BodyPublishers.ofFile(Paths.get("file.json")))
* .build();
* client.sendAsync(request, BodyHandlers.ofString())
* .thenApply(HttpResponse::body)
* .thenAccept(System.out::println); }</pre>
*
* <p> <a id="securitychecks"></a><b>Security checks</b></a>
*
* <p> If a security manager is present then security checks are performed by
* the HTTP Client's sending methods. An appropriate {@link URLPermission} is
* required to access the destination server, and proxy server if one has
* been configured. The form of the {@code URLPermission} required to access a
* proxy has a {@code method} parameter of {@code "CONNECT"} (for all kinds of
* proxying) and a {@code URL} string of the form {@code "socket://host:port"}
* where host and port specify the proxy's address.
*
* @implNote If an explicit {@linkplain HttpClient.Builder#executor(Executor)
* executor} has not been set for an {@code HttpClient}, and a security manager
* has been installed, then the default executor will execute asynchronous and
* dependent tasks in a context that is granted no permissions. Custom
* {@linkplain HttpRequest.BodyPublisher request body publishers}, {@linkplain
* HttpResponse.BodyHandler response body handlers}, {@linkplain
* HttpResponse.BodySubscriber response body subscribers}, and {@linkplain
* WebSocket.Listener WebSocket Listeners}, if executing operations that require
* privileges, should do so within an appropriate {@linkplain
* AccessController#doPrivileged(PrivilegedAction) privileged context}.
*
* @since 11
*/
public abstract class HttpClient {
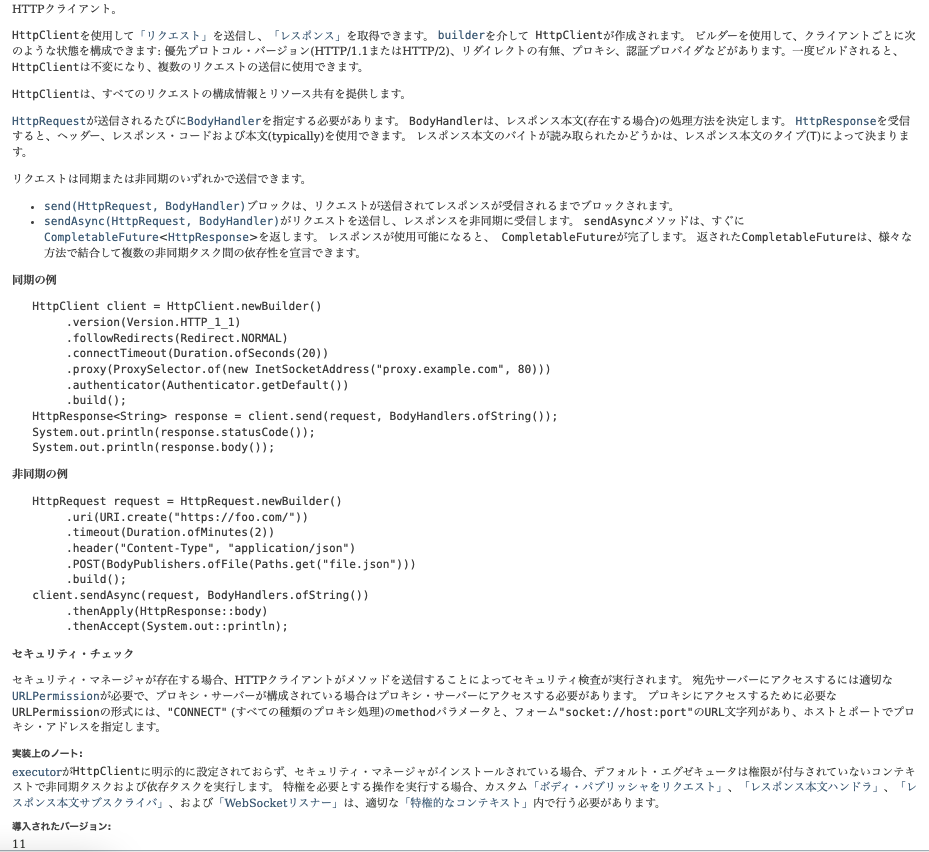
constructor
/**
* Creates an HttpClient.
*/
protected HttpClient() {}
method
メソッドが行うことを記載する。
exceptionや引数・戻り値の注意点も記載する。
/**
* Returns a new {@code HttpClient} with default settings.
*
* <p> Equivalent to {@code newBuilder().build()}.
*
* <p> The default settings include: the "GET" request method, a preference
* of {@linkplain HttpClient.Version#HTTP_2 HTTP/2}, a redirection policy of
* {@linkplain Redirect#NEVER NEVER}, the {@linkplain
* ProxySelector#getDefault() default proxy selector}, and the {@linkplain
* SSLContext#getDefault() default SSL context}.
*
* @implNote The system-wide default values are retrieved at the time the
* {@code HttpClient} instance is constructed. Changing the system-wide
* values after an {@code HttpClient} instance has been built, for
* instance, by calling {@link ProxySelector#setDefault(ProxySelector)}
* or {@link SSLContext#setDefault(SSLContext)}, has no effect on already
* built instances.
*
* @return a new HttpClient
*/
public static HttpClient newHttpClient() {
return newBuilder().build();
}
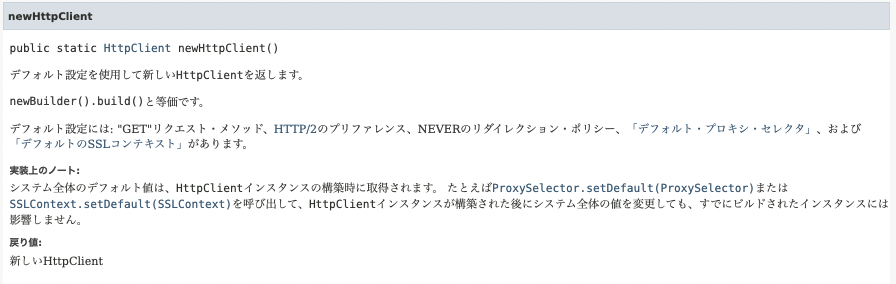
/**
* Sends the given request using this client, blocking if necessary to get
* the response. The returned {@link HttpResponse}{@code <T>} contains the
* response status, headers, and body ( as handled by given response body
* handler ).
*
* @param <T> the response body type
* @param request the request
* @param responseBodyHandler the response body handler
* @return the response
* @throws IOException if an I/O error occurs when sending or receiving
* @throws InterruptedException if the operation is interrupted
* @throws IllegalArgumentException if the {@code request} argument is not
* a request that could have been validly built as specified by {@link
* HttpRequest.Builder HttpRequest.Builder}.
* @throws SecurityException If a security manager has been installed
* and it denies {@link java.net.URLPermission access} to the
* URL in the given request, or proxy if one is configured.
* See <a href="#securitychecks">security checks</a> for further
* information.
*/
public abstract <T> HttpResponse<T>
send(HttpRequest request, HttpResponse.BodyHandler<T> responseBodyHandler)
throws IOException, InterruptedException;
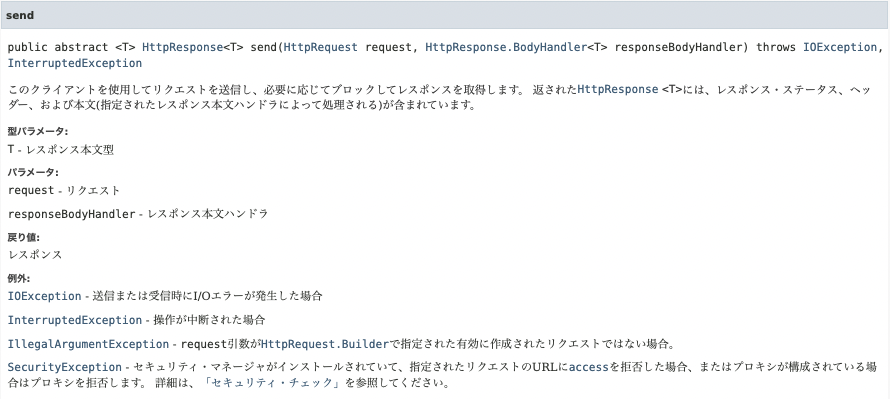
メンバ
メンバが使用される場合の説明を記載する。
定数は特に難しく書く必要はない。
/**
* Defines the automatic redirection policy.
*
* <p> The automatic redirection policy is checked whenever a {@code 3XX}
* response code is received. If redirection does not happen automatically,
* then the response, containing the {@code 3XX} response code, is returned,
* where it can be handled manually.
*
* <p> {@code Redirect} policy is set through the {@linkplain
* HttpClient.Builder#followRedirects(Redirect) Builder.followRedirects}
* method.
*
* @implNote When automatic redirection occurs, the request method of the
* redirected request may be modified depending on the specific {@code 30X}
* status code, as specified in <a href="https://tools.ietf.org/html/rfc7231">
* RFC 7231</a>. In addition, the {@code 301} and {@code 302} status codes
* cause a {@code POST} request to be converted to a {@code GET} in the
* redirected request.
*
* @since 11
*/
public enum Redirect {
/**
* Never redirect.
*/
NEVER,
/**
* Always redirect.
*/
ALWAYS,
/**
* Always redirect, except from HTTPS URLs to HTTP URLs.
*/
NORMAL
}
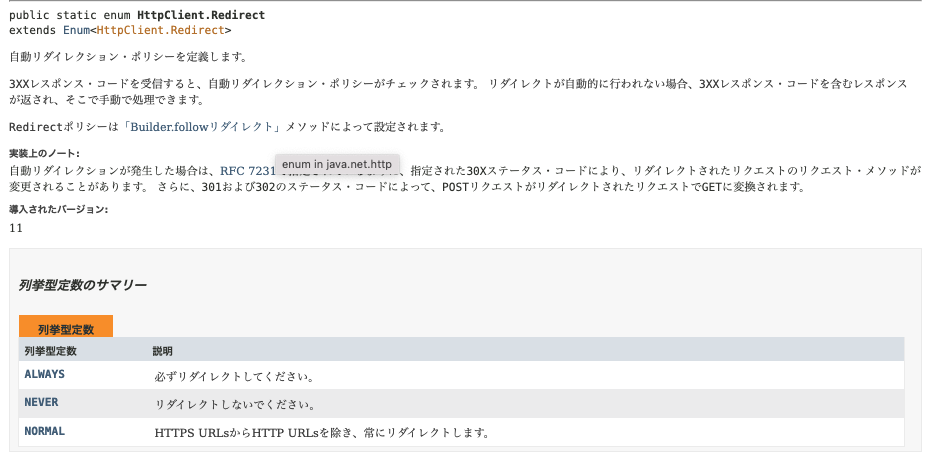